Written by: Stefan Bradstreet
When it comes to developing a web application quickly and can perform well under scale there is no better technology then using python flask outside of NodeJS in terms of speed, development, and flexibility.
In this multipart series I’ll be working on building a web app and highlighting the benefits of flask as a micro framework capable of swapping in and out pieces for various developer needs. This is a multi part blog broken up in terms of discrete functions of code that you’d likely see in a git commit log of a project.
For a development environment I recommend using an Ubuntu virtual machine hosted inside of virtual box. For instructions on doing this check out how to setup and create your own Linux Virtual Machines. I’ll also be using Visual Studio Code as an IDE, other strong recommendation are the Eclipse Web development base with the Python addon, or PyCharm community.
You could also use AWS EC2 as your development environment there which gives you the added benefit of installing gunicorn and nginx for serving the page, and installing and running your own databases. This is a lot of overhead compared to solutions such as Elastic Beanstalk or Heroku which I will be discussing later.
Apple AirPods with Charging Case (Latest Model)
Please help support this site by purchasing items after clicking through our Amazon links
Also, in this blog series we will be setting up an AWS account, creating an IAM user that can talk to a nosql dynamo database, installing the AWS python SDK named BOTO, importing a bootstrap theme, and using the python Jinja framework to manage views.
It sounds like a lot but with some perseverance, patience, and good ol’ grit we can get the job done. Once we get the basic building blocks in place, we can then start adding features to our page such as user access, single sign on from Facebook and Google, messaging, posting, etc…
Once you get your development environment setup in such a way you can run Python, whether it be Windows or the VM solution, next we need to install Flask via PIP. This can be done by running “pip install flask” in a Terminal. Then create a new folder for your project and open this folder in your IDE. Now we should set up our project folder to host a simple page inside our environment.
Let’s create these sub-folders:
- app – app module
- app/controllers – these will host our back end python methods in a modular way
- app/static – this will make up the bulk of the front end web page
- app/templates – this will host one file, index.html , Flask uses this directory to render pages using it’s built in template engine. We could use this as well for doing server side templating which is all well and good but then we don’t get the awesome power of Angular to drive the client side user interface.
Now lets create these files:
- run.py – entry script
- app/__init__.py – tells Python this is the app module and contains logic that runs when the module is bootstrapped by run.py</li>
- app/views.py – this will be our demo view controller, which we will eventually move into the controllers directory as we build out the app
And add the code below to these files
app/__init__.py
# app/__init__.py
from flask import Flask
app = Flask(__name__)
# It's import this after creating app
# so that views can then import app
from app import views
run.py
#!/usr/bin/env python
# Shebang line that will tell the OS to use python to run this script
# Look for a folder named app with a variable named app
# and provide a reference of this object
from app import app
# In __init__ py app is an instance of the Flask object which
# has a run method that will start the web application as a Daemon process
app.run(debug=True)
app/views.py
from app import app
# Decorate the index function with the flask route method
# These methods will be executed when the browser goes to the respective
# routes
@app.route('/')
@app.route('/index')
def index():
return "Hello, World!"
With just these couple of files in place and the skeleton of our app defined we should now be able to locally serve content by running our app.py file for the root project folder. First make sure the script is executable with 755 permissions if you are on a linux operating system and execute it with ./run.py which will serve our page using the localhost address 127.0.0.1 on port 5000. Later on in the project we will host our project on AWS’s elastic beanstalk and use Route57 to assign our page a domain name . From Windows you can run “python run.py” from the python developer I believe. Also by running our application with default=True , the flask framework will monitor our project for changes automatically and restart the internal server for us automatically, how awesome is that!
About Stefan Bradstreet
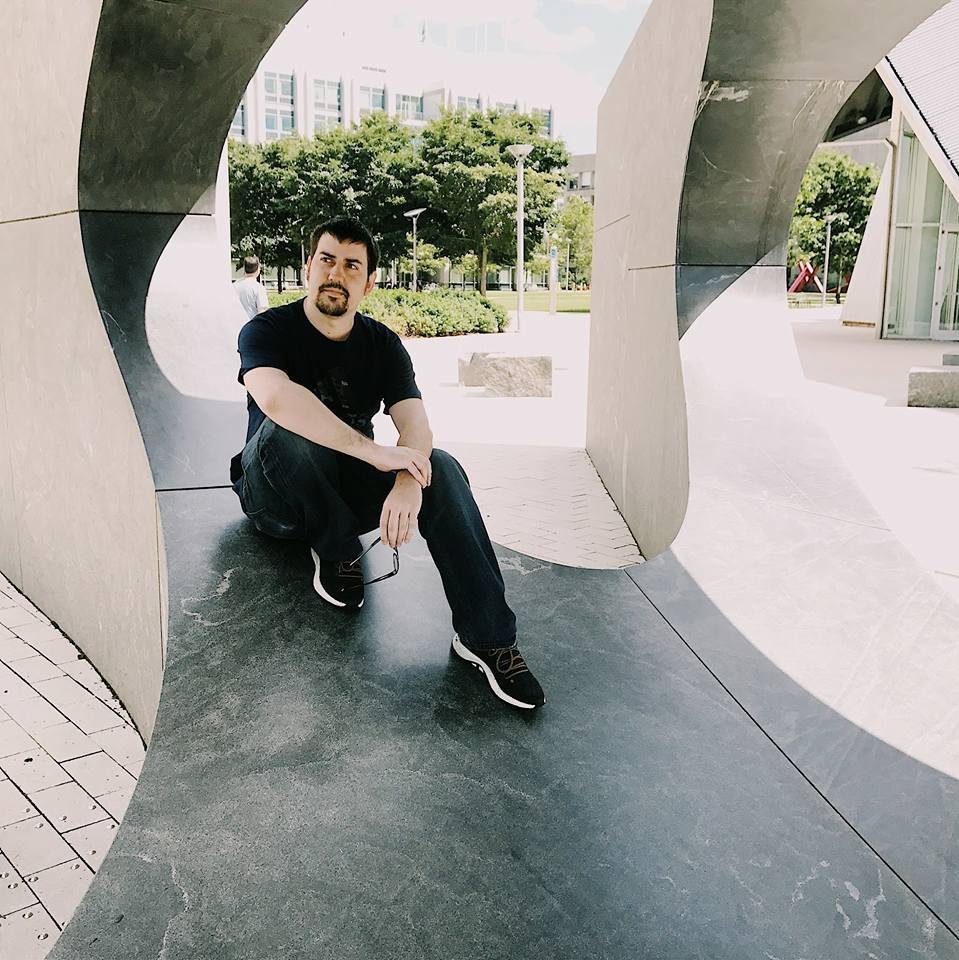
Stefan is a Senior Software Developer at Amazon with 8+ years of experience in tech. He is passionate about helping people become better coders and climbing the ranks in their careers, as well as his own, through continued learning of leadership techniques and software best practices.
One thought on “Creating a Python Web Application using Python Flask”